Python实现的天气时钟
曾经看树莓派实在是可爱,一冲动就买了一个回来,还给它配了外壳和屏幕,然后就这么一直看着它,稀罕但又不知道该拿它来干什么。与其放着吃灰,不如让它发挥点作用,放在桌面上当个时钟吧,而且不仅要能看时间,还要能看天气才行。
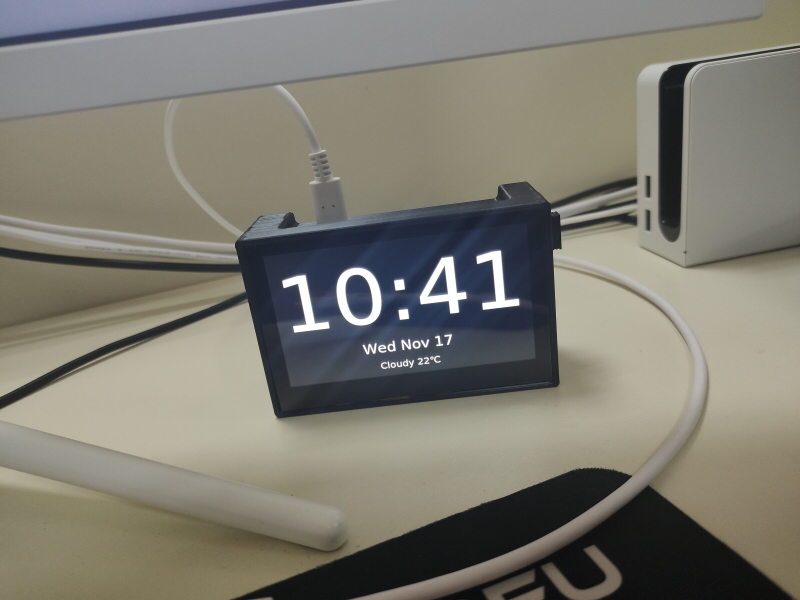
实现过程
使用”time.asctime()”即可获取系统日期和时间。
获取实时天气最简单的方法是调用天气API,这里使用的是心知天气提供的API,如果是个人使用的话免费版就足够了。在控制台查看自己的API密钥,由于是个人使用,使用私钥即可。阅读心知天气产品文档,将示例url中的相应部分替换为自己的私钥,城市和语言。
使用”requests.get(url)”调用天气API,返回的信息可自行筛选,这里只选择了天气和温度。
使用tkinter模块编写图形化界面。
图形化界面的实时刷新功能困扰了我好久,找了各种多进程的方法无果。最后才知道tkinter窗口可以使用after方法实现定时器的作用,让”refresh_time()”,”refresh_date()”,”refresh_weather()”隔一段时间调用自身即可实现刷新。
由于希望这是一个全屏应用,所以树莓派的任务栏会影响观感。编辑树莓派的”~/.config/lxpanel/LXDE-pi/panels/panel”文件,将文件中”autohide=0”改为”autohide=1”,重启,即可隐藏任务栏。
代码
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72
| import requests import time from tkinter import *
root = Tk() root.title('weather clock') root.geometry('800x480') root.config(cursor='none')
frame1 = Frame(root, bg='black') frame1.pack(fill='both')
def get_time(): t = time.asctime() items = t.split(' ') items_time = items[3].split(':') items_time = items_time[0] + ':' + items_time[1]
return items_time
def get_date(): t = time.asctime() items = t.split(' ') items_date = items[0] + ' ' + items[1] + ' ' + items[2]
return items_date
def get_weather(): r = requests.get('https://api.seniverse.com/v3/weather/now.json?key=your_private_key&location=beijing&language=zh-Hans&unit=c') weather = r.json()['results'][0]['now']['text'] + ' ' + r.json()['results'][0]['now']['temperature'] + '℃'
return weather
time_str = StringVar() date_str = StringVar() weather_str = StringVar()
def refresh_time(): global time_str time_str.set(get_time()) root.after(1000, refresh_time)
def refresh_date(): global date_str date_str.set(get_date()) root.after(1000, refresh_date)
def refresh_weather(): global weather_str weather_str.set(get_weather()) root.after(1000 * 60 * 60, refresh_weather)
Label(frame1, textvariable=time_str, bg='black', font=('', 200), fg='white').pack() Label(frame1, textvariable=date_str, bg='black', font=('', 36), fg='white').pack() Label(frame1, textvariable=weather_str, bg='black', font=('', 24), height=2, fg='white').pack()
refresh_time() refresh_date() refresh_weather()
root.mainloop()
|